FAQ ASP.NET/C#
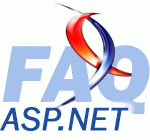
FAQ ASP.NET/C#Consultez toutes les FAQ
Nombre d'auteurs : 39, nombre de questions : 371, dernière mise à jour : 15 juin 2021

Voici une fonction permettant de convertir une valeur en base 10 vers une base n.
private
static
int
increment =
64
;
public
static
string
ToBaseN
(
int
nombre,
int
_base)
{
int
reste;
string
chaine =
string
.
Empty;
while
(
nombre >=
_base)
{
reste =
nombre %
_base;
nombre =
(
nombre -
reste)/
_base;
if
(
reste >
9
)
{
chaine =
((
char
)(
reste -
9
+
increment)).
ToString
(
) +
chaine;
}
else
{
chaine =
reste.
ToString
(
) +
chaine;
}
}
if
(
nombre >
9
)
{
chaine =
((
char
)(
nombre -
9
+
increment)).
ToString
(
) +
chaine;
}
else
{
chaine =
nombre.
ToString
(
) +
chaine;
}
return
chaine.
ToUpper
(
);
}
Conseil: limitez la conversion à la base 36 afin de ne devoir utiliser que les caractères alphanumériques.
Pour mieux comprendre, je vous propose 3 petites étapes qui, au final, vous permettront de traduire sans difficultés une chaine de caractères hexadecimaux; Ceci par l'utilisation notamment de la fonction FromHex de la classe System.Uri
1. Partons du principe qu'un byte est representé par une chaine de 2 caractères hexadecimaux.
Voici deux petites fonctions static verifiant pour nous si la longueur d'une chaine de caractères hexadecimaux est paire ou impaire, puis transformant la chaine hexadecimale en paires de caractères hexadecimaux:
public
static
bool
IsEven
(
string
hexString)
{
return
hexString.
Length %
2
==
0
;
}
public
static
string
[]
ToEvenByteStrings
(
string
hexString)
{
if
(!
IsEven
(
hexString))
{
hexString =
"0"
+
hexString;
}
int
currentChar =
0
;
int
index =
0
;
int
max =
hexString.
Length;
string
[]
byteStrings =
new
string
[
Convert.
ToInt32
(
max /
2
) -
1
];
while
(
currentChar <
max)
{
byteStrings
(
index) =
hexString.
Substring
(
currentChar,
2
);
index +=
1
;
currentChar +=
2
;
}
return
byteStrings;
}
2. la fonction Uri.FromHex retourne la valeur décimale d'un caractère hexadécimal.
Utilisons-la donc pour nous retourner un byte pour une chaine de 2 caractères hexadecimaux:
public
static
byte
ToByteFromHex
(
string
byteString)
{
byte
byte1 =
Convert.
ToByte
(
Uri.
FromHex
(
byteString[
0
]
));
byte
byte2 =
Convert.
ToByte
(
Uri.
FromHex
(
byteString[
1
]
));
return
Convert.
ToByte
((
byte1 *
16
) +
byte2);
}
3. Il nous reste à present à implementer la fonction qui nous retournera un tableau de byte quelquesoit la longueur de la chaine hexadecimale
public
static
byte
[]
BytesFromHex
(
string
hexString)
{
byte
[]
bytes =
null
;
int
max =
hexString.
Length;
if
(
max >
0
)
{
if
(
max ==
1
)
{
bytes =
new
byte
[]{
ToByteFromHex
(
"0"
+
hexString)};
}
else
{
int
index =
0
;
string
[]
byteStrings =
ToEvenByteStrings
(
hexString);
bytes =
new
byte
[
byteStrings.
Length -
1
];
foreach
(
string
byteString in
byteStrings)
{
bytes
(
index) =
ToByteFromHex
(
byteString);
index +=
1
;
}
}
}
return
bytes;
}
Lors de l'utilisation de BytesFromHex, il faudra juste veiller à la possibilité d'un retour de valeur null en cas de chaine vide passée en paramètre.
Lien : Méthode Uri.FromHex
La solution est de créer une instance du type désiré. Pour savoir le type réel de l'objet, la méthode GetType() est de grande utilité. Ensuite, on charge l'Assembly "Forms" pour enfin modifier le Type de l'instance.
static
public
Control ObjectToControl
(
object
obj)
{
string
typeName =
obj.
GetType
(
).
ToString
(
);
Assembly a =
Assembly.
GetAssembly
(
typeof
(
Form));
Type t =
a.
GetType
(
typeName);
Control c =
(
Control)Activator.
CreateInstance
(
t);
c =
(
Control)Convert.
ChangeType
(
obj,
t);
return
c;
}
Voici quelques fonctions static qui vous permettront de convertir tout numerique entier en son equivalent chaine de caractères hexadécimaux, simplement en utilisant une surcharge de ToString() et le format X.
Transformation effective pour les types byte, UInt16, UInt32, UInt64
public
static
string
ToHexString
(
byte
byte
)
{
return
byte
.
ToString
(
"X2"
,
null
);
}
public
static
string
ToHexString
(
UInt16 uint16)
{
return
uint16.
ToString
(
"X4"
,
null
);
}
public
static
string
ToHexString
(
UInt32 uint32)
{
return
uint32.
ToString
(
"X8"
,
null
);
}
public
static
string
ToHexString
(
UInt64 uint64)
{
return
uint64.
ToString
(
"X16"
,
null
);
}
Il ne vous reste qu'à prevoir la conversion pour les types restant SByte, short, int, long :
public
static
string
ToHexString
(
SByte sbyte
)
{
return
ToHexString
(
Convert.
ToByte
(
sbyte
));
}
public
static
string
ToHexString
(
short
int16)
{
return
ToHexString
(
Convert.
ToUInt16
(
int16));
}
public
static
string
ToHexString
(
int
int32)
{
return
ToHexString
(
Convert.
ToUInt32
(
int32));
}
public
static
string
ToHexString
(
long
int64)
{
return
ToHexString
(
Convert.
ToUInt64
(
int64));
}