FAQ ASP.NET/VB.NET
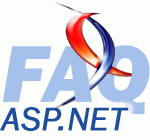
FAQ ASP.NET/VB.NETConsultez toutes les FAQ
Nombre d'auteurs : 38, nombre de questions : 369, dernière mise à jour : 16 juin 2021

Voici une fonction permettant de convertir une valeur en base 10 vers une base n.
Private
Shared
increment As
Integer
=
64
Public
Shared
Function
ToBaseN
(
ByVal
nombre As
Integer
, ByVal
_base As
Integer
) As
String
Dim
reste As
Integer
Dim
chaine As
String
=
String
.Empty
While
nombre >=
_base
reste =
nombre Mod
_base
nombre =
(
nombre -
reste) /
_base
If
reste >
9
Then
chaine =
CType
((
reste -
9
+
increment), Char
).ToString
+
chaine
Else
chaine =
reste.ToString
+
chaine
End
If
End
While
If
nombre >
9
Then
chaine =
CType
((
nombre -
9
+
increment), Char
).ToString
+
chaine
Else
chaine =
nombre.ToString
+
chaine
End
If
Return
chaine.ToUpper
End
Function
Conseil: limitez la conversion à la base 36 afin de ne devoir utiliser que les caractères alphanumériques.
Pour mieux comprendre, je vous propose 3 petites étapes qui, au final, vous permettront de traduire sans difficultés une chaine de caractères hexadecimaux; Ceci par l'utilisation notamment de la fonction FromHex de la classe System.Uri
1. Partons du principe qu'un byte est representé par une chaine de 2 caractères hexadecimaux.
Voici deux petites fonctions static verifiant pour nous si la longueur d'une chaine de caractères hexadecimaux est paire ou impaire, puis transformant la chaine hexadecimale en paires de caractères hexadecimaux:
Public
Shared
Function
IsEven
(
ByVal
hexString As
String
) As
Boolean
If
(
hexString.Length
Mod
2
=
0
) Then
Return
True
Else
Return
False
End
Function
Public
Shared
Function
ToEvenByteStrings
(
ByVal
hexString As
String
) As
String
(
)
If
Not
IsEven
(
hexString) Then
hexString =
"0"
&
hexString
Dim
currentChar As
Integer
=
0
Dim
index As
Integer
=
0
Dim
max As
Integer
=
hexString.Length
Dim
byteStrings
(
) As
String
=
New
String
(
Convert.ToInt32
(
max /
2
) -
1
) {}
While
(
currentChar <
max)
byteStrings
(
index) =
hexString.Substring
(
currentChar, 2
)
index +=
1
currentChar +=
2
End
While
Return
byteStrings
End
Function
2. la fonction Uri.FromHex retourne la valeur décimale d'un caractère hexadécimal.
Utilisons-la donc pour nous retourner un byte pour une chaine de 2 caractères hexadecimaux:
Public
Shared
Function
ToByteFromHex
(
ByVal
byteString As
String
) As
Byte
Dim
byte1 As
Byte
=
Convert.ToByte
(
Uri.FromHex
(
byteString.Chars
(
0
)))
Dim
byte2 As
Byte
=
Convert.ToByte
(
Uri.FromHex
(
byteString.Chars
(
1
)))
Return
Convert.ToByte
((
byte1 *
16
) +
byte2)
End
Function
3. Il nous reste à present à implementer la fonction qui nous retournera un tableau de byte quelquesoit la longueur de la chaine hexadecimale
Public
Shared
Function
BytesFromHex
(
ByVal
hexString As
String
) As
Byte
(
)
Dim
bytes
(
) As
Byte
=
Nothing
Dim
max As
Integer
=
hexString.Length
If
(
max >
0
) Then
If
(
max =
1
) Then
: bytes =
New
Byte
(
) {ToByteFromHex
(
"0"
&
hexString)}
Else
Dim
index As
Integer
=
0
Dim
byteStrings
(
) As
String
=
ToEvenByteStrings
(
hexString)
Dim
byteString As
String
bytes =
New
Byte
(
byteStrings.Length
-
1
) {}
For
Each
byteString In
byteStrings
bytes
(
index) =
ToByteFromHex
(
byteString)
index +=
1
Next
End
If
End
If
Return
bytes
End
Function
Lors de l'utilisation de BytesFromHex, il faudra juste veiller à la possibilité d'un retour de valeur null en cas de chaine vide passée en paramètre.
Lien : Méthode Uri.FromHex
La solution est de créer une instance du type désiré. Pour savoir le type réel de l'objet, la méthode GetType() est de grande utilité. Ensuite, on charge l'Assembly "Forms" pour enfin modifier le Type de l'instance.
Public
Shared
Function
ObjectToControl
(
ByVal
obj As
Object
) As
Control
Dim
typeName
As
String
=
obj.GetType.ToString
Dim
a As
Assembly
=
Assembly
.GetAssembly
(
GetType
(
Form))
Dim
t As
Type
=
a.GetType
(
typeName
)
Dim
c As
Control =
CType
(
Activator.CreateInstance
(
t), Control)
c =
CType
(
Convert.ChangeType
(
obj, t), Control)
Return
c
End
Function
Voici quelques fonctions static qui vous permettront de convertir tout numerique entier en son equivalent chaine de caractères hexadécimaux, simplement en utilisant une surcharge de ToString() et le format X.
Transformation effective pour les types byte, UInt16, UInt32, UInt64
Public
Shared
Function
ToHexString
(
ByVal
[byte
] As
Byte
) As
String
Return
[byte
].ToString
(
"X2"
, Nothing
)
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[uint16] As
UInt16) As
String
Return
[uint16].ToString
(
"X4"
, Nothing
)
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[uint32] As
UInt32) As
String
Return
[uint32].ToString
(
"X8"
, Nothing
)
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[uint64] As
UInt64) As
String
Return
[uint64].ToString
(
"X16"
, Nothing
)
End
Function
Il ne vous reste qu'à prevoir la conversion pour les types restant SByte, short, int, long :
Public
Shared
Function
ToHexString
(
ByVal
[sbyte
] As
SByte
) As
String
Return
ToHexString
(
Convert.ToByte
(
[sbyte
]))
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[int16] As
Short
) As
String
Return
ToHexString
(
Convert.ToUInt16
(
[int16]))
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[int32] As
Integer
) As
String
Return
ToHexString
(
Convert.ToUInt32
(
[int32]))
End
Function
Public
Shared
Function
ToHexString
(
ByVal
[int64] As
Long
) As
String
Return
ToHexString
(
Convert.ToUInt64
(
[int64]))
End
Function